Download and parse map data¶
Download OpenStreetMap data¶
You can use the following two methods to download the OpenStreetMap data:
1. OpenStreetMapOfficial:
choose Export
-> OverpassAPI
(otherwise the size of the bounding box size is limited).
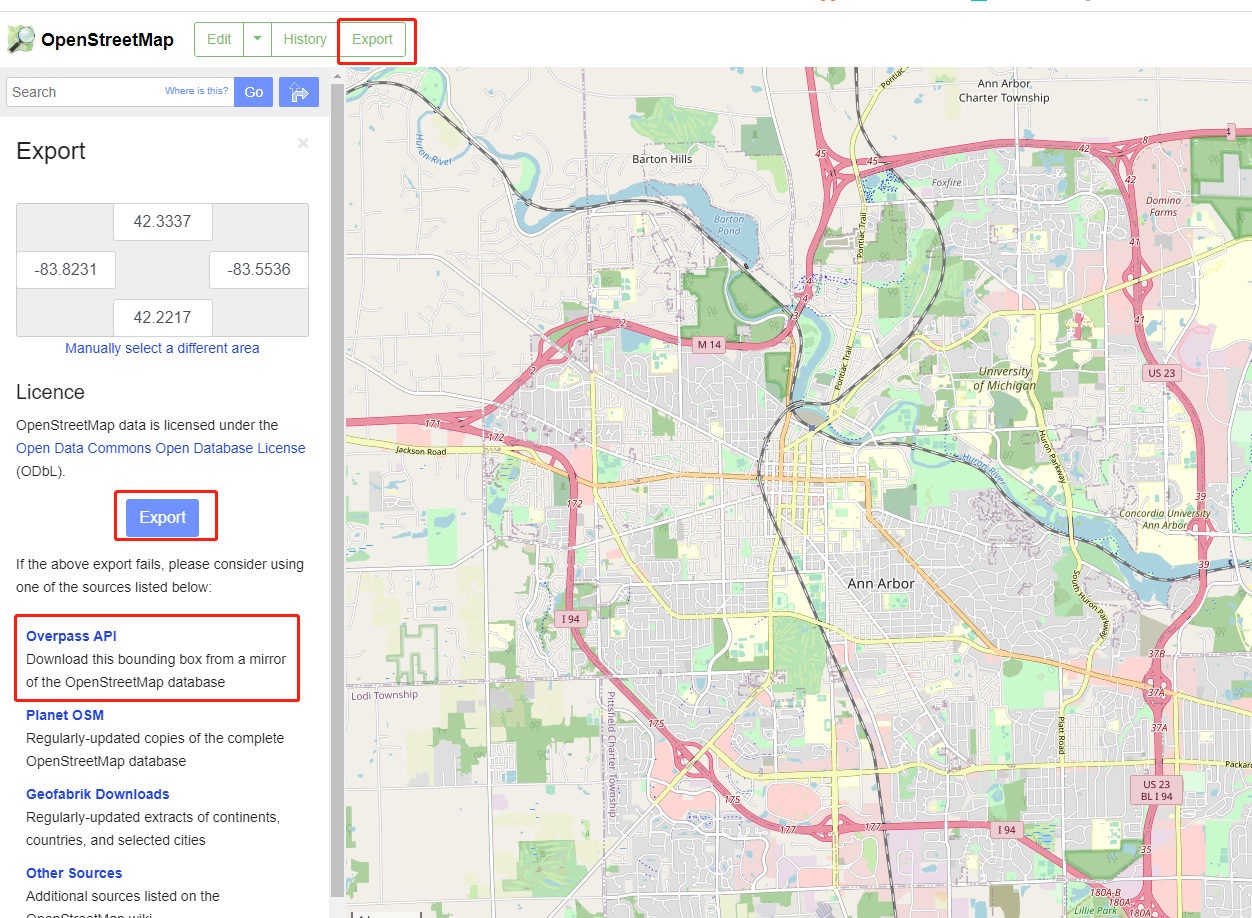
By this way, you will get the complete OSM data (xml),
then you can use osm_filter.osm_way_filter()
to get
the layer you need.
2. Overpass API (recommended): directly use overpass API to download the layer you need. The sample query code is attached in the appendix Appendix A: Query code.
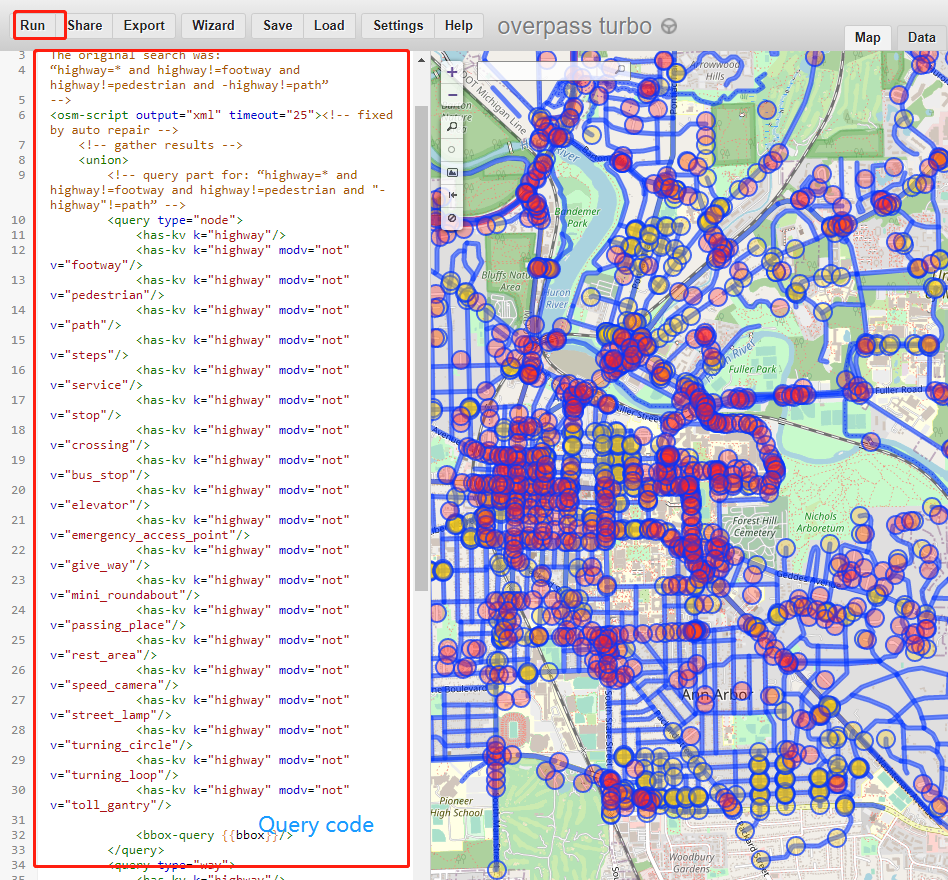
In this way, you will directly get the layer you need.
Parse OpenStreetMap data¶
The downloaded OpenStreetMap xml file can be parsed using
the mtldp.mtlmap.build_network_from_xml()
:
1 2 | import mtldp.mtlmap as mtlmap
network = mtlmap.build_network_from_xml("*.osm")
|
This will return you a well-structured class
mtldp.mtlmap.Network
.
Appendix A: Query code¶
Here is an example of the query code using the OverpassAPI.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 | <!--
This has been generated by the overpass-turbo wizard.
The original search was:
“highway=* and highway!=footway and highway!=pedestrian and -highway!=path”
-->
<osm-script output="xml" timeout="25"><!-- fixed by auto repair -->
<!-- gather results -->
<union>
<!-- query part for: “highway=* and highway!=footway and highway!=pedestrian and "-highway"!=path” -->
<query type="node">
<has-kv k="highway"/>
<has-kv k="highway" modv="not" v="footway"/>
<has-kv k="highway" modv="not" v="pedestrian"/>
<has-kv k="highway" modv="not" v="path"/>
<has-kv k="highway" modv="not" v="steps"/>
<has-kv k="highway" modv="not" v="service"/>
<has-kv k="highway" modv="not" v="stop"/>
<has-kv k="highway" modv="not" v="crossing"/>
<has-kv k="highway" modv="not" v="bus_stop"/>
<has-kv k="highway" modv="not" v="elevator"/>
<has-kv k="highway" modv="not" v="emergency_access_point"/>
<has-kv k="highway" modv="not" v="give_way"/>
<has-kv k="highway" modv="not" v="mini_roundabout"/>
<has-kv k="highway" modv="not" v="passing_place"/>
<has-kv k="highway" modv="not" v="rest_area"/>
<has-kv k="highway" modv="not" v="speed_camera"/>
<has-kv k="highway" modv="not" v="street_lamp"/>
<has-kv k="highway" modv="not" v="turning_circle"/>
<has-kv k="highway" modv="not" v="turning_loop"/>
<has-kv k="highway" modv="not" v="toll_gantry"/>
<bbox-query {{bbox}}/>
</query>
<query type="way">
<has-kv k="highway"/>
<has-kv k="highway" modv="not" v="footway"/>
<has-kv k="highway" modv="not" v="pedestrian"/>
<has-kv k="highway" modv="not" v="path"/>
<has-kv k="highway" modv="not" v="steps"/>
<has-kv k="highway" modv="not" v="service"/>
<bbox-query {{bbox}}/>
</query>
<query type="relation">
<has-kv k="highway"/>
<has-kv k="highway" modv="not" v="footway"/>
<has-kv k="highway" modv="not" v="pedestrian"/>
<has-kv k="highway" modv="not" v="path"/>
<has-kv k="highway" modv="not" v="steps"/>
<has-kv k="highway" modv="not" v="service"/>
<bbox-query {{bbox}}/>
</query>
</union>
<!-- print results -->
<print mode="meta"/><!-- fixed by auto repair -->
<recurse type="down"/>
<print mode="meta" order="quadtile"/><!-- fixed by auto repair -->
</osm-script>
|
Appendix B: Network classes¶
Network¶
mtldp.mtlmap.Network
is the highest level
class that includes all different kinds of information of links,
segments, lane sets, and nodes defined blow.
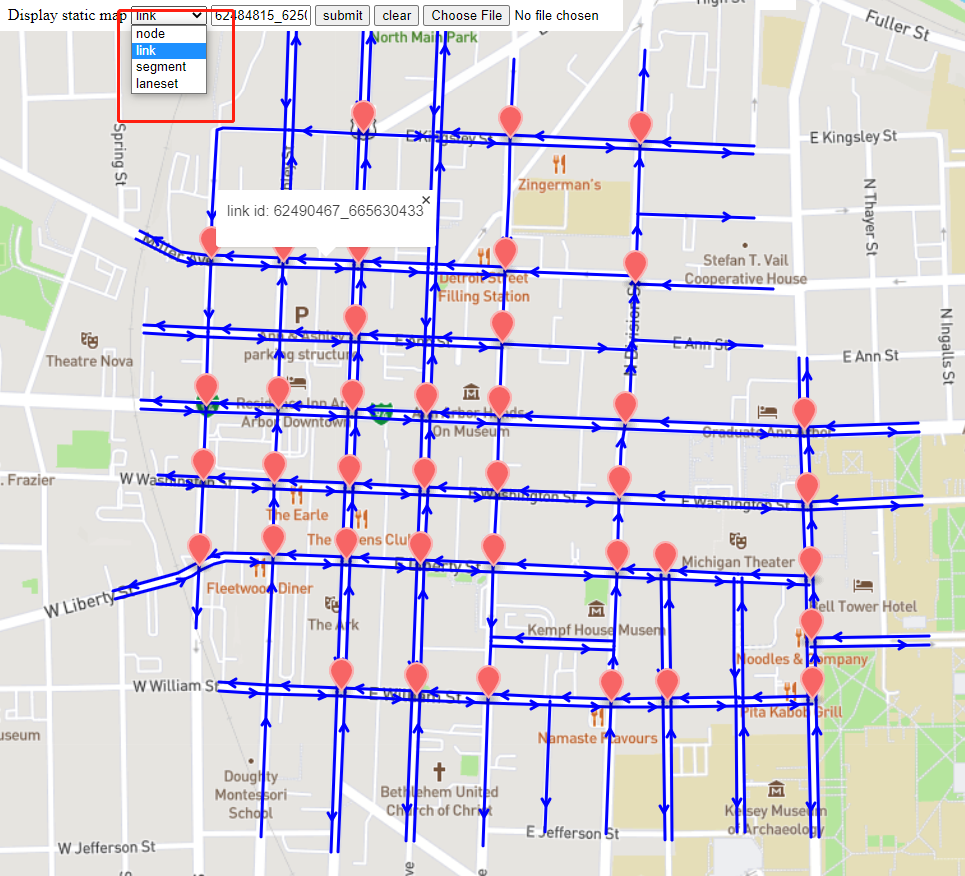
Node¶
Node is parsed from the node
in the OpenStreetMap data.
Established based on a general mtldp.mtlmap.Node
class that
contains essential attributes including node id, GPS coordinates,
we further divide the nodes into different categories including
mtldp.mtlmap.SegmentConnectionNode
, mtldp.mtlmap.SignalizedNode
,
mtldp.mtlmap.UnSignalizedNode
, and mtldp.mtlmap.EndNode
.
In the figure below, the red mark denotes a signalized node.

Link¶
mtldp.mtlmap.Link
is defined as a road segment composed of a series of segments that
connects two signalized nodes, and it includes direction information.
The blue arrows in the figure below denote the links between
two nodes.
Note
All the road segments including Link
, Segment
, LaneSet
are directed.

Segment¶
mtldp.mtlmap.Segment
is used to define a road segment that has the uniform
tags and parameters (speed limit, lane number and usage, etc.). Each segment is directly
generated from a way
from the OpenStreetMap data (we also have a
class mtldp.mtlmap.OsmWay
that is directly parsed from the OpenStreetMap data).
In the figure below, the blue arrows
denote the segments. There might be multiple segments between
two nodes.

LaneSet¶
mtldp.mtlmap.LaneSet
class denotes a set of lanes that has the same downstream direction
(e.g. through, right turn, left turn).
The blue arrows in the figure below denote the lane sets.
For example, the blue arrows that are connected to the left
intersection represent the through movement and left turn
movement.
